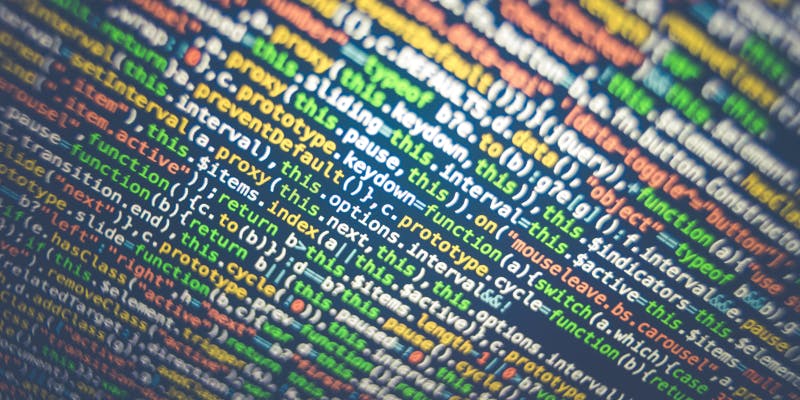
(Recap) ES5 to ES6+
Back in 2015, almost six years after the release of the previous version of ECMAScript we got our hands on ES6. Theres a lot of great stuff in this set which help us to write cleaner and more manageable code. It was a pretty big deal and so I'm going to recap some of the more useful or interesting additions it brought to us.
Proper Default Parameters
No longer did we need to use || to have default values and worry about what would happen if we needed zero as a default.
function(height = 50, color = 'red');
As a side note, unlike some other languages, default parameters can come before those without defaults in ES6.
Destructuring Assignment
This one can be a little harder to follow than some of the other changes. It looks a little bit like magic. This lets you pull variables or objects out of other objects by name, something like this:
const {username, password} = req.body
Here the request body contains a username and password. Before we would have to assign "req.body.{param}" to a variable for both individually. Now we can just pull them out directly and use them as is.
Arrow Functions
One of the most anticipated changes to come into the language. Arrow functions fix the way 'this' behaves, at least inside the arrow function itself. It's not that magic. Thanks to this, gone are the days where we needed 'that = this' and to some degree '.bind(this)'. And like much of the other changes it can shorten our code quite nicely.
const incrementA = a => a + 1; const sum = (a, b) => { return a + b; }
With a single parameter, single line function you no longer needed parens at all and the return is implicit. No more need for the function keyword either.
Block-Scoped Constructs - Let and Const
This is not a sugarcoating feature. It’s more intricate. let is a new version of var which allows us to scope the variable to the block. We define blocks by the curly braces. In ES5, blocks had no affect on our variables.
function calculateTotalAmount (extra) { var amount = 0 // should also be let, but you can mix var and let if (extra) { // This is its own scope let amount = 1 // first amount is still 0 } { // another scope let amount = 100 // first amount is still 0 { // and another scope let amount = 1000 // first amount is still 0 } } return amount // returns 0 }
Since all the declarations of amount after the first are using let within a different block scope they have no affect to the functions scope. So amount never changes.
Const is also block scoped but as it implies is read-only, in that while the value can change the reference must remain the same.
This is by no means all the changes and it doesn't go into any particular depth, but it's a good reminder to refresh our knowledge every now and then. Despite using these and more on a potentially daily basis, we can all forget things. Something else we should remember, these changes are mostly just syntactic sugar on top of the JS of old. And so, while it's great that it's improving and we get all these fancy new features, it is important not to forget how it used to work. What ES6 looks like under the hood.